예제는 『Java Design Patterns A Tour of 23 Gang of Four Design Patterns in Java』 (Apress, 2016) 에서 가지고 옴
추상클래스가 객체 생성을 정의하지만, 객체 생성은 하위 서브클래스가 결정하는 구조.
JDK에서 사용된 예 - SocketFactory
https://docs.oracle.com/javase/8/docs/api/index.html?javax/net/SocketFactory.html
■ UML Class Diagram
IAnimalFactory : Animal 생성을 정의한 추상 클래스
ConcreteFactory : 객체 생성 담당, IAnimalFactory의 구현(하위) 클래스, AnimalType을 인자로 받아서 객체를 생성한다
IAnimal : Animal 정의 인터페이스
Duck, Tiger : IAnimal 구현한 클래스
Animal을 사용하는 입장에서는 어떤 타입의 Animal이 리턴되더라도 IAnimal 인터페이스를 구현하였으므로 speak() 메서드가 실행된다. 유연성을 부여한 클래스 구조
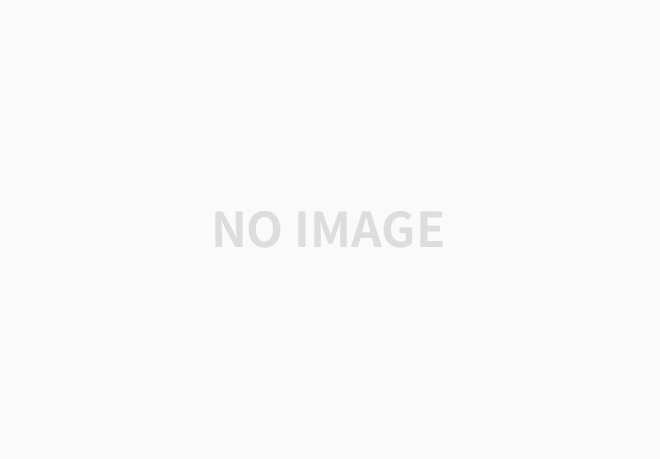
■ Code Snippet
abstract class IAnimalFactory
{
public abstract IAnimal GetAnimalType(String type) throws Exception; //if we cannot instantiate later we'll throw exception
}
class ConcreteFactory extends IAnimalFactory
{
@Override
public IAnimal GetAnimalType(String type) throws Exception
{
switch (type)
{
case "Duck":
return new Duck();
case "Tiger":
return new Tiger();
default:
throw new Exception( "Animal type : "+type+" cannot be instantiated");
}
}
}
class FactoryPatternEx
{
public static void main(String[] args) throws Exception
{
System.out.println("***Factory Pattern Demo***\n");
IAnimalFactory animalFactory = new ConcreteFactory();
IAnimal DuckType=animalFactory.GetAnimalType("Duck");
DuckType.Speak();
IAnimal TigerType = animalFactory.GetAnimalType("Tiger");
TigerType.Speak();
//There is no Lion type. So, an exception will be thrown
IAnimal LionType = animalFactory.GetAnimalType("Lion");
LionType.Speak();
}
}
√ 실행 결과
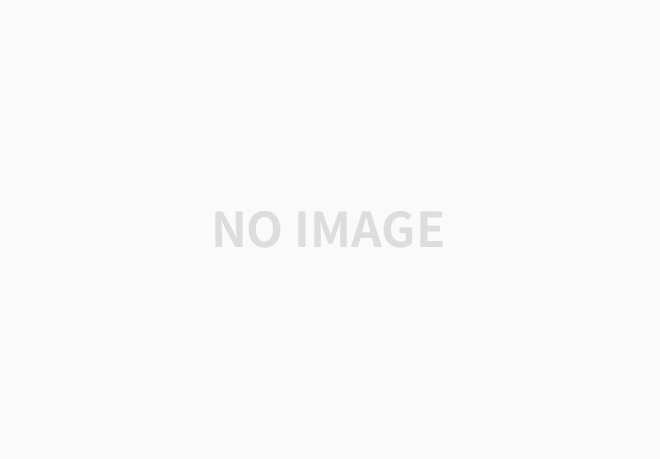
■ 정리
1) 객체 생성을 하위 클래스로 옮길 때 유용하다
2) 병렬 클래스 계층을 구현할 때 또는 느슨한 결합인 시스템을 만들 때 유용하다.
3) 너무 많은 객체를 만들면 성능이 저하될 수 있다.
※ 참고 자료
1) 『Java Design Patterns A Tour of 23 Gang of Four Design Patterns in Java』 (Apress, 2016)
2) Example Source
https://github.com/apress/java-design-patterns
'DEV' 카테고리의 다른 글
Design Patterns - 4. Adapter Pattern (0) | 2021.10.24 |
---|---|
Design Patterns - 3. Singleton Pattern (0) | 2021.10.06 |
Design Patterns - 1. 디자인 패턴이란 (0) | 2021.10.06 |
Scouter - 설치 (0) | 2021.09.28 |
GitLab - 프로젝트에 멤버 추가하기 (0) | 2020.04.14 |